Author |
Help with the trail code... |
Faustus Marshal Palestar

Joined: May 29, 2001 Posts: 2748 From: Austin, Texas
| Posted: 2004-02-05 17:36  
Here is the code that renders the trails for the ships... firstly, I've fixed most of the render issues with the ship/missile trails. However, I'd like to use only 1 triangle strip to render the entire trail, currently I'm pushing 2 crossed triangle strips which isn't exactly the greatest solution...
For those of you who might be 3D gurus, please take a look at the following code... the vector vY needs to be set so that the trail appears facing the viewer at all times.
void Trail::renderTrail( RenderContext &context, const Matrix33 & frame,
const Vector3 & position, const Vector3 & vHead )
{
if (! m_TrailMaterial.valid() )
return;
// simple visiblity check
if (! context.sphereVisible( position, m_TrailRadius ) )
return;
int nTrailSize = m_Trail.size();
int nVerts = (nTrailSize + 1) * 2;
if ( nVerts < 4 )
return;
DisplayDevice * pDisplay = context.display();
ASSERT( pDisplay );
// create the triangle strip primitive
PrimitiveTriangleStripDL::Ref pStrip = PrimitiveTriangleStripDL::create( pDisplay, nVerts );
// lock the primitive
VertexL * pVert = pStrip->lock();
const Matrix33 mWorldFrame( context.frame() );
const Vector3 vNormal( 0, 0, 0 );
const Vector3 vY( 0, m_TrailMaterial->height(), 0 );
const Color cDiffuse( 255,255,255,s_BaseAlpha );
// get the head position in view space
Vector3 vHeadVS( mWorldFrame * (vHead - context.position()) );
pVert->position = vHeadVS + vY;
pVert->normal = vNormal;
pVert->diffuse = cDiffuse;
pVert->u = 0.0f;
pVert->v = 0.0f;
pVert++;
pVert->position = vHeadVS - vY;
pVert->normal = vNormal;
pVert->diffuse = cDiffuse;
pVert->u = 0.0f;
pVert->v = 1.0f;
pVert++;
// calculate the maximum life of a segment, this is used to calculate the UV coordinates for the segments
const float fMaxLife = Max( s_TrailScalar * m_TrailLife, 1.0f );
// start at the end, since thats actually the beginning of the trail
m_Trail.end();
while( m_Trail.valid() )
{
Segment & segment = *m_Trail;
m_Trail.prev();
// get the UV coordinates for this segment
const float fT = float( segment.life ) / fMaxLife;
const float fU = Clamp( 1.0f - fT, 0.05f, 0.95f );
const Color cDiffuse( 255, 255, 255, fT * s_BaseAlpha );
// get the viewspace position of this segment
const Vector3 vSegmentVS( mWorldFrame * (segment.position - context.position()) );
pVert->position = vSegmentVS + vY;
pVert->normal = vNormal;
pVert->diffuse = cDiffuse;
pVert->u = fU;
pVert->v = 0.0f;
pVert++;
pVert->position = vSegmentVS - vY;
pVert->normal = vNormal;
pVert->diffuse = cDiffuse;
pVert->u = fU;
pVert->v = 1.0f;
pVert++;
}
pStrip->unlock();
// set the material
Material::push( context, m_TrailMaterial );
// set the transform line strip from worldspace to viewspace
PrimitiveSetTransform::createPush( pDisplay, Matrix33( true ), Vector3( true ) );
// push the triangle strip
PrimitiveTriangleStripDL::push( pDisplay, pStrip );
}
_________________
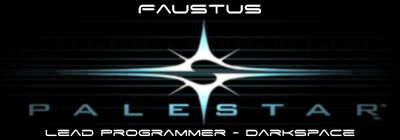
|
milo Cadet
Joined: February 06, 2004 Posts: 1
| Posted: 2004-02-06 01:31  
Hi Faustus,
Someone sent me a link to this thread. If you are just looking for a way to calculate vY, here is the equivalent code from my game engine:
Point head = trail[1] + loc;
Point tail = trail[0] + loc;
Point vcam = camview->Pos() - head;
Point vtmp = vcam.cross(head-tail);
vtmp.Normalize();
Point vlat = vtmp * (width + (0.1 * width * ntrail));
verts->loc[0] = tail - vlat;
verts->loc[1] = tail + vlat;
My "vlat" is your "vY'. Depending on your conventions, you may need to change the signs to ensure that the polygon faces directly at the camera instead of directly away from it.
Basically, the idea is to take a vector from the trail to the camera position and then compute the cross-product of that with a vector that is parallel to the length of the trail. The result of the cross-product is always perpendicular to both input vectors. This guarantees that the resulting polygon is always normal to the camera.
Q.E.D.
--milo
http://www.starshatter.com
P.S. A good place to go get these kinds of questions answered is the Microsoft DirectX Developers Mailing List. At least, it used to be great for that; I haven't been there in years. Here's a link to the web based archive - http://discuss.microsoft.com/SCRIPTS/WA-MSD.EXE?A0=directxdev&D=1
[ This Message was edited by: milo on 2004-02-06 01:35 ]
_________________
|
Dwarden Admiral CHIMERA
Joined: June 07, 2001 Posts: 1072 From: Czech Republic
| Posted: 2004-02-06 07:48  
Nice to see Milo here ... really suprising moment ...
_________________ ... Ideas? ... that's Ocean w/o borders !
|
Gideon Cadet
Joined: September 14, 2001 Posts: 4604 From: Oregon, USA
| Posted: 2004-02-06 10:51  
Starshatter!
WOOT!!! One of the other games that's been taking up all my time! I love it, and it's not even complete yet! (Using public beta version.)
_________________ ...and lo, He looked upon His creation, and said, "Fo shizzle."
|
lilFrigate (Marys Soldier) Vice Admiral
Joined: August 30, 2002 Posts: 92 From: Police Station
| Posted: 2004-02-06 19:26  
milo! long time no see, i havent checked out starshatter for a while, which is when you posted it on the bc forums...
whoever sent you the link did a good job.
_________________ For what does it prophet a man if he gaineth the whole world but loseth his soul?
Equilibrium!
|